How to Create a Wordle Game & Word Cloud | Complete Guide
You can create a Wordle game using HTML, CSS, and JavaScript, or use online tools like mywordle.strivemath.com, puzzel.org, or thewordfinder.com for custom word puzzles without coding.
What is Wordle?
Wordle has become a cultural phenomenon that captures the attention of word enthusiasts worldwide. Understanding what Wordle is provides the foundation for creating your own version.
The Word Guessing Game
Wordle is a popular word puzzle game where players attempt to guess a five-letter word within six tries. After each guess, the game provides feedback by coloring the letters: green indicates a correct letter in the correct position, yellow shows a correct letter in the wrong position, and gray means the letter isn’t in the target word. This simple yet addictive game was created by Josh Wardle and later acquired by The New York Times.
The game’s popularity stems from its simplicity, the satisfaction of solving the puzzle, and the social aspect of sharing results. The limited number of daily puzzles creates a shared experience among players worldwide.
The Word Cloud Generator
The term “Wordle” also refers to a word cloud generator that creates visual representations of text. In this application, words appear in different sizes based on their frequency in the source text. The more frequently a word appears, the larger it displays in the visualization.
Word clouds serve as valuable tools for text analysis, highlighting key themes and prominent words within a body of text. Educators often use word clouds to summarize content, visualize feedback, or demonstrate language patterns in literature.
Creating Wordle Using Online Tools
For those who prefer not to code from scratch, several online platforms offer user-friendly interfaces to create custom Wordle games.
Using mywordle.strivemath.com
Mywordle.strivemath.com provides a straightforward way to create custom Wordle puzzles. The platform allows you to set your own word for friends, students, or family to solve.
To create a Wordle on this platform:
- Visit mywordle.strivemath.com
- Select your preferred language from the options
- Enter your chosen word in the designated field
- Click the “Generate Link” button
- Share the generated link with the intended players
This platform maintains the classic Wordle gameplay while allowing customization of the target word. Players receive the same letter-color feedback system as the original game, making it familiar and accessible.
Using thewordfinder.com
The Word Finder offers a robust Wordle maker with additional customization options. Their tool allows for words between 3 and 9 letters, expanding beyond the traditional 5-letter limitation.
The process to create a custom Wordle on thewordfinder.com includes:
- Enter your chosen word in the main text box
- Wait for the puzzle to generate automatically
- Use the “Send Game” button to share via social media or email
- Players can then enjoy the game with the same rules as standard Wordle
The platform also offers educational features, including the ability to learn more about the answer word after completing the puzzle. This makes it particularly useful for vocabulary building and language learning applications.
Using puzzel.org
Puzzel.org provides another free online Wordle generator with unique features. This platform allows you to create Wordles of any length and even add multiple words for players to solve in sequence.
Key features of the puzzel.org Wordle maker include:
- Freedom to choose words of any length
- Option to create a series of words as a multi-puzzle challenge
- Real-time word validation to prevent random guessing
- Support for special characters like ü, á, and ê
- Option to add media descriptions for context or hints
- Celebratory confetti animation upon successful completion
The platform focuses on user-friendly design, with a programmatic keyboard that works well on mobile devices without requiring the mobile keyboard to appear, keeping gameplay clean and focused.
Creating Wordle from Scratch with Code
For developers or those wanting to learn coding, building a Wordle game from scratch offers a rewarding project that teaches fundamental web development concepts.
Setting Up the HTML Structure
The HTML structure forms the skeleton of your Wordle game. It creates the visual elements players interact with, including the game board and input fields.
A basic HTML structure for a Wordle game includes:
xml<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Custom Wordle Game</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<h1>Wordle Game</h1>
<div id="gameBoard"></div>
<input type="text" id="guessInput" maxlength="5">
<button id="guessButton">Guess</button>
<script src="script.js"></script>
</body>
</html>
This structure creates a title, a game board div that will contain the letter cells, an input field for guesses, a submission button, and connects the CSS and JavaScript files needed for styling and functionality.
Adding CSS for Styling
CSS styling transforms the basic HTML structure into an attractive, user-friendly game interface. The styling defines colors, layout, and responsive behavior.
A sample CSS implementation for the Wordle game might look like:
cssbody {
font-family: Arial, sans-serif;
text-align: center;
background-color: #f0f0f0;
}
#gameBoard {
display: grid;
grid-template-columns: repeat(5, 1fr);
gap: 10px;
margin: 20px auto;
max-width: fit-content;
}
.cell {
width: 60px;
height: 60px;
border: 2px solid #333;
text-align: center;
line-height: 60px;
font-size: 2em;
}
.cell.correct {
background: #538d4e;
color: white;
}
.cell.semi-correct {
background: #b59f3b;
color: white;
}
.cell.incorrect {
background: #3a3a3c;
color: white;
}
@media (max-width: 400px) {
.cell {
width: 40px;
height: 40px;
border: 1px solid #333;
text-align: center;
line-height: 40px;
font-size: 1em;
}
}
This CSS creates a grid layout for the game board, styles the individual letter cells, defines colors for correct, partially correct, and incorrect guesses, and includes responsive design for mobile devices.
Creating the Game Logic with JavaScript
JavaScript provides the functionality that makes the game interactive. It handles user input, checks guesses against the target word, and provides feedback.
A simplified version of the JavaScript code might include:
javascriptconst gameBoard = document.getElementById('gameBoard');
const guessInput = document.getElementById('guessInput');
const guessButton = document.getElementById('guessButton');
const inputs = [];
const results = [];
let targetWord = 'WORDLE'; // The word players need to guess
// Initialize game
function initializeGame() {
for (let i = 0; i < 30; i++) { // 6 attempts, 5 letters each
let cell = document.createElement('div');
cell.classList.add('cell');
let y = Math.floor(i / 5);
let x = i - y * 5;
if(inputs.length > y && inputs[y][x]) {
const result = results[y][x] === "+" ? "correct" :
results[y][x] === "x" ? "semi-correct" : "incorrect";
cell.textContent = inputs[y][x].toUpperCase();
cell.classList.add(result);
}
gameBoard.appendChild(cell);
}
}
// Check guess against target word
function checkGuess(guess) {
const result = [];
const word = targetWord.toLowerCase();
guess = guess.toLowerCase();
for (let i = 0; i < guess.length; i++) {
if (guess[i] === word[i]) {
result.push("+"); // Correct position
} else if (word.includes(guess[i])) {
result.push("x"); // In word but wrong position
} else {
result.push("-"); // Not in word
}
}
return result;
}
// Update game board display
function refreshGame() {
gameBoard.innerHTML = '';
initializeGame();
}
// Event listener for the guess button
guessButton.addEventListener('click', function() {
let guess = guessInput.value.toUpperCase();
if (guess.length === 5) {
inputs.push(guess);
results.push(checkGuess(guess));
guessInput.value = "";
refreshGame();
// Check if player won
if (guess === targetWord) {
setTimeout(() => {
alert("Congratulations! You guessed the word!");
}, 300);
} else if (inputs.length === 6) {
setTimeout(() => {
alert("Game over! The word was " + targetWord);
}, 300);
}
} else {
alert('Please enter a 5-letter word.');
}
});
initializeGame();
This code creates the game board, handles player guesses, checks them against the target word, provides visual feedback, and manages win/loss conditions.
Implementing the Wordle Algorithm
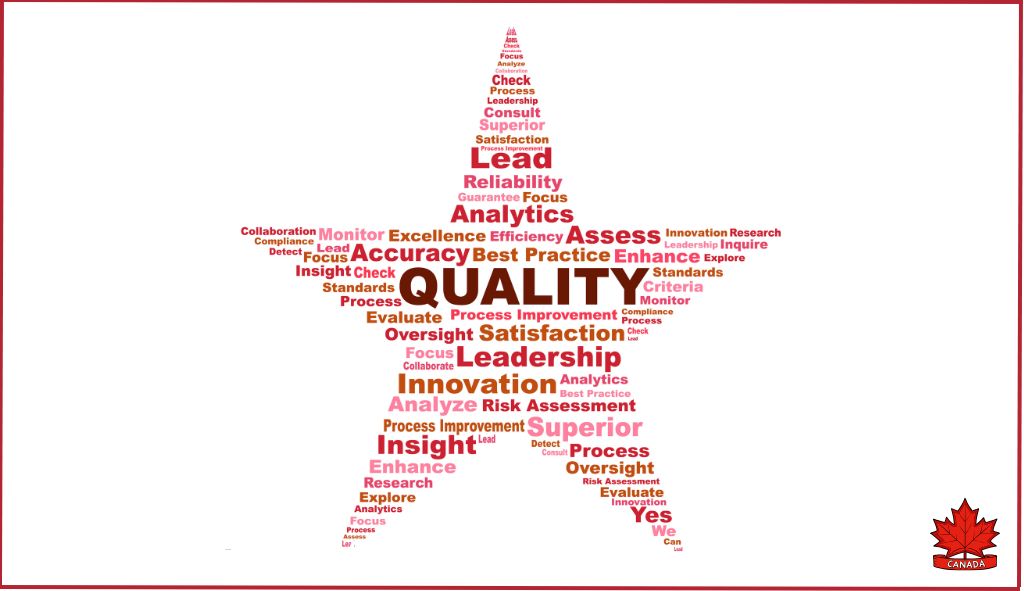
The core of Wordle’s gameplay lies in its algorithm for checking guesses and providing feedback. A more sophisticated implementation might include:
- Handling duplicate letters correctly
- Validating words against a dictionary
- Generating random words for players to guess
For a production-quality game, you might use an API to validate words and generate random words. Some developers incorporate the Wordle Game API or create their own word validation system.
A more advanced implementation might look like:
javascriptfunction validateGuess(guess) {
return fetch('https://api.dictionaryapi.dev/api/v2/entries/en/' + guess)
.then(response => {
if (response.ok) {
return true; // Valid English word
} else {
return false; // Not a valid word
}
})
.catch(error => {
console.error('Error checking word:', error);
return true; // Default to accepting the word if API fails
});
}
async function handleGuess() {
let guess = guessInput.value.toLowerCase();
if (guess.length === 5) {
const isValid = await validateGuess(guess);
if (isValid) {
// Process the valid guess
} else {
alert('Not a valid English word. Try again.');
}
} else {
alert('Please enter a 5-letter word.');
}
}
This code demonstrates how you might validate guesses against a dictionary API before accepting them, adding another layer of challenge to the game.
Adding Animations and Transitions
Animations make the game feel more dynamic and provide visual feedback that enhances player engagement. You can add CSS animations to reveal the results of each guess:
css.cell {
transition: all 0.3s ease;
transform-style: preserve-3d;
}
.cell.flip {
animation: flip 0.5s ease forwards;
}
@keyframes flip {
0% {
transform: rotateX(0);
}
50% {
transform: rotateX(90deg);
}
100% {
transform: rotateX(0);
}
}
Pair this CSS with JavaScript to trigger the animations when a player submits a guess:
javascriptfunction animateResults(row) {
const cells = document.querySelectorAll('.row-' + row + ' .cell');
cells.forEach((cell, index) => {
setTimeout(() => {
cell.classList.add('flip');
}, index * 100); // Stagger the animation
});
}
These animations create a satisfying reveal process as each letter’s result is shown, similar to the official Wordle game.
User Feedback and Error Handling
Clear communication with players improves the gaming experience. Implement feedback mechanisms for different scenarios:
- Invalid words (not in dictionary)
- Incorrect word length
- Game completion (win or loss)
- Letter frequency information
You can use simple alerts or create more sophisticated toast notifications:
javascriptfunction showNotification(message, type = 'info') {
const notification = document.createElement('div');
notification.classList.add('notification', type);
notification.textContent = message;
document.body.appendChild(notification);
setTimeout(() => {
notification.classList.add('show');
}, 10);
setTimeout(() => {
notification.classList.remove('show');
setTimeout(() => {
notification.remove();
}, 300);
}, 2000);
}
With corresponding CSS:
css.notification {
position: fixed;
top: 20px;
left: 50%;
transform: translateX(-50%);
padding: 10px 20px;
background-color: #333;
color: white;
border-radius: 5px;
opacity: 0;
transition: opacity 0.3s ease;
z-index: 1000;
}
.notification.show {
opacity: 1;
}
.notification.error {
background-color: #e74c3c;
}
.notification.success {
background-color: #2ecc71;
}
This creates pop-up notifications that appear when needed and automatically disappear after a short delay.
Storing Game Data
Implementing data storage allows players to continue games across sessions and keeps track of statistics.
Using Local Storage
The browser’s localStorage API provides a simple way to save game data:
javascriptfunction saveGameState() {
const gameState = {
targetWord: targetWord,
inputs: inputs,
results: results,
gameStatus: gameStatus
};
localStorage.setItem('wordleGameState', JSON.stringify(gameState));
}
function loadGameState() {
const savedState = localStorage.getItem('wordleGameState');
if (savedState) {
const gameState = JSON.parse(savedState);
targetWord = gameState.targetWord;
inputs = gameState.inputs;
results = gameState.results;
gameStatus = gameState.gameStatus;
refreshGame();
}
}
Call saveGameState()
after each guess and loadGameState()
when the page loads to maintain continuity between sessions.
Reset and Continue Game Options
Provide players with options to manage their game:
javascriptfunction resetGame() {
inputs = [];
results = [];
gameStatus = 'playing';
targetWord = getRandomWord(); // Function to get a new random word
localStorage.removeItem('wordleGameState');
refreshGame();
}
// Add button event listeners
document.getElementById('resetButton').addEventListener('click', resetGame);
Include UI elements that allow players to:
- Start a new game with a different word
- Share their results
- View game statistics
- Access game instructions
These options enhance replay value and user engagement.
Making the Game Challenging
A compelling Wordle game needs the right level of challenge to keep players engaged.
Implementing a Dictionary of Words
Instead of hardcoding a single word, create or incorporate a dictionary of possible target words:
javascriptconst wordList = [
'APPLE', 'BRAVE', 'CACTUS', 'DANCE', 'EAGLE',
'FLAME', 'GLOBE', 'HOUSE', 'IMAGE', 'JOKER',
// Add hundreds more words
];
function getRandomWord() {
const randomIndex = Math.floor(Math.random() * wordList.length);
return wordList[randomIndex];
}
For a more comprehensive solution, you might load a larger word list from an external file or API:
javascriptasync function loadWordList() {
try {
const response = await fetch('wordlist.json');
const data = await response.json();
return data.words;
} catch (error) {
console.error('Error loading word list:', error);
return defaultWordList; // Fallback to a smaller built-in list
}
}
This approach provides variety and prevents players from seeing repeated words.
Adding Difficulty Levels and Daily Challenges
Implement different difficulty levels to accommodate players of varying skill:
javascriptfunction setDifficulty(level) {
switch(level) {
case 'easy':
wordList = easyWords; // Shorter, more common words
maxAttempts = 8; // More attempts allowed
break;
case 'normal':
wordList = normalWords;
maxAttempts = 6;
break;
case 'hard':
wordList = hardWords; // Longer, less common words
maxAttempts = 5; // Fewer attempts
break;
}
resetGame();
}
You can also implement a daily challenge feature that gives all players the same word on a given day:
javascriptfunction getDailyWord() {
const today = new Date();
const dateString = `${today.getFullYear()}-${today.getMonth()+1}-${today.getDate()}`;
const dateHash = hashString(dateString); // Create a consistent hash from the date
const wordIndex = dateHash % wordList.length; // Use the hash to pick a word
return wordList[wordIndex];
}
function hashString(str) {
let hash = 0;
for (let i = 0; i < str.length; i++) {
hash = ((hash << 5) - hash) + str.charCodeAt(i);
hash |= 0; // Convert to 32bit integer
}
return Math.abs(hash);
}
This creates a consistent word selection based on the date, ensuring all players get the same challenge on the same day.
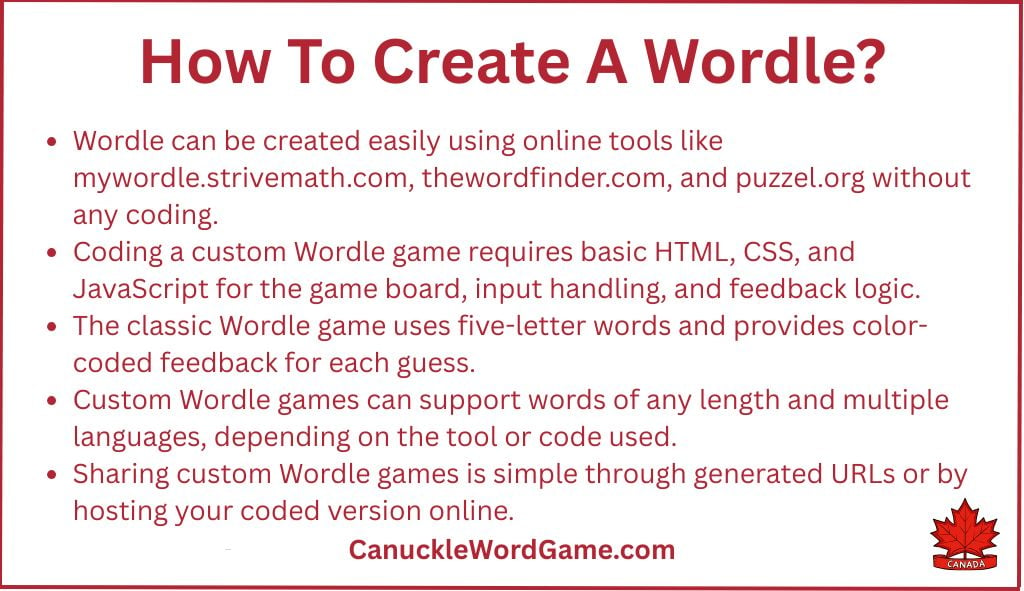
Educational Applications of Wordle
Wordle has significant educational potential beyond entertainment.
Using Wordle in Education
Educators can use custom Wordle games to:
- Reinforce vocabulary in language classes
- Test subject-specific terminology
- Create engaging review activities
- Support language development for English learners
For example, a science teacher might create a Wordle with biology terms, while a language teacher might focus on irregular verbs or thematic vocabulary.
The interactive nature of Wordle makes it an effective tool for active learning, encouraging students to apply their knowledge in a game-based context.
Benefits of Creating Wordle for Learning
Creating custom Wordle games offers several educational benefits:
- Vocabulary reinforcement: Players must recall and spell words correctly
- Pattern recognition: The feedback system teaches players to identify letter patterns
- Deductive reasoning: Players use elimination and logical thinking to narrow down possibilities
- Engagement: The game format increases student motivation and participation
- Adaptive learning: Custom Wordles can target specific learning objectives and student needs
Research suggests that game-based learning tools like Wordle can increase student engagement and improve knowledge retention by making the learning process more enjoyable and interactive.
Tips and Best Practices
Follow these guidelines to create an effective Wordle game.
Design Considerations
When designing your Wordle game, consider:
- Color accessibility: Use high-contrast colors and consider color-blind friendly alternatives (patterns or symbols in addition to colors)
- Mobile responsiveness: Ensure your game works well on all device sizes
- Keyboard layout: Provide an on-screen keyboard for easier input, especially on mobile
- Clear instructions: Include a brief tutorial or help section for new players
- Loading states: Add visual indicators when the game is processing (especially when checking against a dictionary API)
The visual design should be clean and focused, avoiding cluttered interfaces that might distract from the core gameplay.
Performance Optimization
Optimize your Wordle game with these techniques:
- Minimize API calls: Cache validated words to reduce server requests
- Efficient DOM manipulation: Update only the elements that need to change
- Debounce input handling: Prevent excessive function calls during fast typing
- Preload assets: Load word lists and other resources at startup
- Minify code: Reduce file sizes for production deployment
These optimizations ensure smooth gameplay even on slower devices or connections.
Frequently Asked Questions
What is the difference between Wordle and a word cloud?
Wordle refers to both a word-guessing game and a word cloud generator. The word-guessing game challenges players to identify a hidden word through feedback on letter placement. A word cloud is a visual representation of text data where word size correlates with frequency in the source text.
How many letters should a Wordle word contain?
Traditional Wordle uses five-letter words, but custom versions can use words of any length. For educational purposes, word length should match learning objectives and student ability levels. When creating your own Wordle, consider your audience’s vocabulary level.
Can I create a Wordle in languages other than English?
Yes, many Wordle creators support multiple languages. When coding your own Wordle, you can implement any language by providing an appropriate word list and accounting for special characters or accents. Online platforms like mywordle.strivemath.com and puzzel.org offer multi-language support.
How do I share my custom Wordle with others?
Most online Wordle makers generate a unique URL for your custom game that you can share via email, social media, or messaging apps. If you’ve coded your own Wordle, you’ll need to host it on a web server and share that URL.
What makes a good Wordle word?
Good Wordle words are recognizable to your target audience, have a mix of common and uncommon letters, and present a moderate challenge. Avoid obscure words unless creating for educational purposes with a specific vocabulary focus. Words with repeated letters create an interesting challenge.